You (Might) Only Need a Microcontroller (and a Server) for Computer Science
Introduction
I recently read a blog post that got me nerdsnipped. The blog post was: You Only Need a Tablet for Computer Science. In here, Ezri talks about the setup they use. An iPad and a server. Using the tablet just to connect to a server. That got me thinking. What is the least amount of power you need to connect to an actual server? Enter the ESP8266.
The ESP8266 is a microcontroller that can connect to the internet and has the following specs:
My ESP8266 is fancy, I have 4MiB of Flash.
- Processor: L106 32-bit RISC microprocessor core based on the Tensilica Diamond Standard 106Micro running at 80 or 160 MHz.
- Memory: 160KiB of RAM total.
- 32 KiB instruction RAM
- 32 KiB instruction cache RAM
- 80 KiB user-data RAM
- 16 KiB ETS system-data RAM
- External QSPI flash: up to 16 MiB is supported (512 KiB to 4 MiB typically included)
As per wikipedia.
I bought a couple of this badboys last year for my final project of the microcontrollers class. I had them lying around and thought that it would be fun to use them to connect to my computer.
Connecting to my computer
I decided that I wanted to do a simple proof of concept to demonstrate that it is possible to connect from a micro to my computer. The ESP8266 is powerfull enough to run something like an SSH client, right?
After a swift fifteen minutes of searching, all I could find was a library that implemented SSH servers and clients for the ESP32. But I did not want to port an entire library just for a nerdsnipe.
I decided that I should use something a bit older, telnet!
Disclaimer: telnet makes all your communications in plain text so you should not use it for anything serious. But I am not making something serious.
There is a port of an Arduino™ library for ESP32 to ESP8266 that almost works.
I imported the library to the arduino™ IDE and when I tried compiling the example. I got some compilation errors.
A quick fix of two lines got this running.
-#include "ESP8266telnetClient.h"
+#include "ESP8266TelnetClient.h
and
bool ESP8266telnetClient::sendCommand(const char* cmd){
this->send(cmd);
//negotiation until the server show the command prompt again
if (strcmp(cmd, "exit") != 0){
return this->waitPrompt();
}
else{
this->disconnect();
+ return false; // not even sure if this is should work lol
}
}
I made a telnet server on my computer and I ran the example code, it ran. I had successfully run a telnet client from this Microprocessor.
But the example code only had hard coded commands and I wanted a more interactive shell. So I decided to code something simple up and modify the example. I made a kind of interactive shell with the help of chatgpt:
void setup() {
// Wifi setup...
.setPromptChar('$');
tc
char key = 0;
.println("\r\nPress Enter to begin:");
Serialdo {
= Serial.read();
key } while (key <= 0);
if (tc.login(laptop, user, pwd)) {
.sendCommand("ls -l");
tc.sendCommand("ifconfig");
tc= true; // Set interactive shell flag to true
interactiveShellActive (); // Call the interactive shell function
interactiveShell= false; // Reset the interactive shell flag
interactiveShellActive }
else {
.println("Login failed");
Serial}
}
void loop() {
}
void interactiveShell() {
.println("Interactive Shell. Type 'exit' to end.");
Serialwhile (true) {
//Serial.print("Enter command: ");
= readSerialStringUntil('\n');
String command
if (command == "exit") {
break;
}
.sendCommand(command.c_str());
tc}
}
(char delimiter) {
String readSerialStringUntil= "";
String inputString while (Serial.available() > 0) {
char c = Serial.read();
if (c == delimiter || c == '\n') {
break;
}
+= c;
inputString }
return inputString;
}
Finally, I ran this and had an interactive shell through telnet! There is a problem with this code though, the shell keeps printing again and again and again. But, it’s a full terminal!
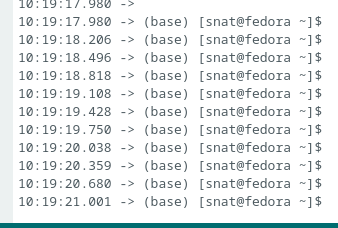
You can only communicate with this terminal one line at a time. So no VIM in this shell :(. Buuuut, you still have ed!
So you can write stuff like:
$ ed
a
Hello, World!
.
wq
Conclusion
You can absolutely do better than this. It was a fun tangent for a day. For now, I rest my case. You only need a microcontroller with a total of 160KiB of RAM to go to university.
If you want to read the code, it is in my repo with the “patched” library m.
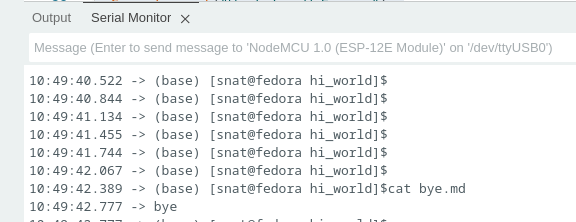